Piano
The Piano
Sonic Pi plays all piano keys. The command play 70
means the 70th key, play 71
plays the next to the right. Sonic Pi plays also decimal notes, try play 71.67
. Also, you can play multiple notes at the time:
play 72
play 75
play 79
Melody
Use sleep
command in between.
s = 0.5
play 72
sleep s
play 75
sleep s
play 79
Notes
The notes C, D, E, F, G, A, B
are available. Also, the black keys are there; use s
as sharp or b
as flat.
play :C
sleep 0.5
play :D
sleep 0.5
play :E
The different octaves are available as numbers, try play :C3
or play :Fs3
.
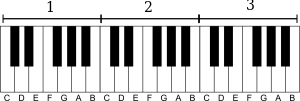
Chords
Chords are usually written on top of the charts.
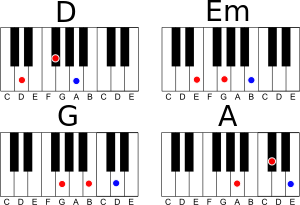
play chord(:D, :minor7)
sleep 1
play chord(:D, :major7)
sleep 1
play chord(:D, "7")
sleep 1
play chord(:Db, "7")
sleep 1
play chord(:Db, :major7)
sleep 1
play chord(:E3, :minor)
sleep 1
play chord(:E3, :major)
sleep 1
play chord(:E, "7")
sleep 1
play chord(:G, :minor7)
sleep 1
play chord(:A, :major)
sleep 1
play chord(:G, "m7-5")
Also, you can type the chord in once: play_chord [:c4, :e4, :g4]
. More chords are available at e.g. Chord progression map.
Variables
Play all the keys starting from the 60th key.
nappain = 60
live_loop :kaikki do
play nappain, attack:0.01, sustain:0.1, release:0.9
sleep 1.5
nappain = nappain + 1
end
Functions
The Jingle Bells is a very famous Christmas song. Note that there is a lot of repeating, and thus we code the lines in own functions:
use_bpm 70
define :line1 do
play :E
sleep 0.5
play :E
sleep 0.5
play :E
sleep 0.5
play :E
sleep 0.5
play :E
sleep 0.5
play :E
sleep 0.5
play :E
sleep 0.5
play :G
sleep 0.5
play :C
sleep 0.5
play :D
sleep 0.5
play :E
sleep 0.5
end
define :line2 do
play :F
sleep 0.5
play :F
sleep 0.5
play :F
sleep 0.5
play :F
sleep 0.5
play :F
sleep 0.5
play :E
sleep 0.5
play :E
sleep 0.5
play :E
sleep 0.5
end
define :line3 do
play :E
sleep 0.5
play :D
sleep 0.5
play :D
sleep 0.5
play :E
sleep 0.5
play :D
sleep 0.5
play :G
end
define :line4 do
play :G
sleep 0.5
play :G
sleep 0.5
play :F
sleep 0.5
play :D
sleep 0.5
play :C
sleep 0.5
end
line1
line2
line3
line1
line2
line4
The lines can be written shorter as
use_bpm 70
define :line1 do
play_pattern_timed [:e, :e, :e, :e, :e, :e], [0.5, 0.5, 0.5, 0.5, 0.5, 0.5]
sleep 0.5
play_pattern_timed [:e, :g, :c, :d, :e], [0.5, 0.5, 0.5, 0.5, 0.5]
sleep 0.5
end
define :line2 do
play_pattern_timed [:f, :f, :f, :f, :f, :e, :e, :e], [0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5]
sleep 0.5
end
define :line3 do
play_pattern_timed [:e, :d, :d, :e, :d, :g], [0.5, 0.5, 0.5, 0.5, 0.5]
end
define :line4 do
play_pattern_timed [:g, :g, :f, :d, :c], [0.5, 0.5, 0.5, 0.5, 0.5]
sleep 0.5
end
line1
line2
line3
line1
line2
line4
Modifying notes
Mods: Volume, Amplification
play 60, amp: 0.5
sleep 1
play 60, amp: 2
Mods: Stereo
play 60, pan: -1
sleep 1
play 60, pan: 1
sleep 1
play 60, pan: 0
Mods: Release time
play 70, release: 1
sleep 1
play 70, release: 2
sleep 2
play 70, release: 0.2
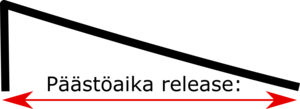
Mods: Attack time
play 60, attack: 2
sleep 3
play 65, attack: 0.5
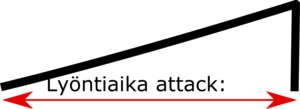
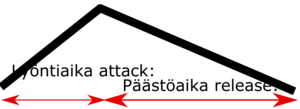
Mods: Sustain time
play 60, attack: 0.3, sustain: 1, release: 1
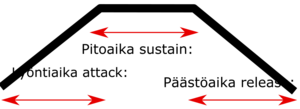
Mods: Decay time and level
play 60, attack: 0.1, attack_level: 1, decay: 0.2, sustain_level: 0.4, sustain: 1, release: 0.5
play 60, attack: 0.1, attack_level: 1, decay: 0.2, decay_level: 0.3, sustain: 1, sustain_level: 0.4, release: 0.5
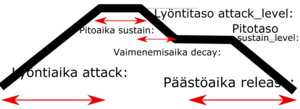
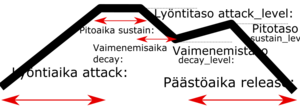