Raspberry Pi: Difference between revisions
Line 224: | Line 224: | ||
First start the Python script on the Raspberry Pi and then run the EV3 code. | First start the Python script on the Raspberry Pi and then run the EV3 code. | ||
[[File:BT ev3g hello.png|thumb| | [[File:BT ev3g hello.png|thumb|Multiple hellos.]] | ||
<syntaxhighlight lang="python"> | <syntaxhighlight lang="python"> |
Revision as of 22:33, 1 November 2020
Setting Up The Raspian
Establish Wifi, Bluetooth.
Credentials are pi@raspberrypi.local
and raspberry
. KiTTY seems to be a good terminal.
Set Up WiFi without Screen
Into root directory:
- Add an empty file called
ssh
- Add a file
wpa_supplicant.conf
which includes
country=ee
update_config=1
ctrl_interface=/var/run/wpa_supplicant GROUP=netdev
network={
scan_ssid=1
ssid="YOUR_NETWORK_NAME"
psk="YOUR_PASSWORD"
key_mgmt=WPA-PSK
}
Note the (Notepad++: Edit) EOL conversion UNIX.
https://www.raspberrypi-spy.co.uk/2017/04/manually-setting-up-pi-wifi-using-wpa_supplicant-conf/
USB cable // Perhaps requires Zero
Connect via usb to gadget mode. After flashing RaspianOS. The following files are at boot
-directory
- Modify
config.txt
: adddtoverlay=dwc2
- Add an empty file called
ssh
- Edit
cmdline.txt
: Ensure that there existrootwait modules-load=dwc2,g_ether
.
Now you can ssh pi@raspberrypi.local
if the usb cable is plugged in.
Bluetooth on Raspi 3
sudo apt update
sudo apt upgrade
sudo apt-get install python-bluez
#sudo apt install bluetooth pi-bluetooth bluez blueman
sudo nano /etc/systemd/system/dbus-org.bluez.service
. Modify ExecStart=/usr/lib/bluetooth/bluetoothd –C
sudo sdptool add SP
sudo reboot
sudo apt-get install bluetooth bluez libbluetooth-dev
sudo python3 -m pip install pybluez
Raspi and VIM
sudo apt install vim
sudo apt install vim-nox
#Vundle
git clone https://github.com/VundleVim/Vundle.vim.git ~/.vim/bundle/Vundle.vim
vim ~/.vimrc
" Syntax highlight
syntax enable
" Tabs are spaces
set expandtab
" Number of spaces per tab
set tabstop=2
" Search as soon as characters are entered
set incsearch
" Highlight search results
set hlsearch
https://stackoverflow.com/questions/18948491/running-python-code-in-vim
https://scribles.net/enabling-python-autocomplete-in-vim-on-raspberry-pi/
Bundle 'Valloric/YouCompleteMe'
Raspi and Ev3-G
Error Messages so far
raspberry pi device added succesfuly but failed to connect
Connection failed: _blueman.RFCOMMError: Failed to get rfcomm channel
Paired successfully but this device has no services which can be used with raspberry pi
Pairing ev3 and Raspi
Pairing and connecting is easy using X11.
bluetooth-agent
command do not exist on Jessie. Use bluetoothctl
with the following script shown on Stackechange:
sudo bluetoothctl <<EOF
power on
discoverable on
pairable on
agent NoInputNoOutput
default-agent
EOF
bluetoothctl
power on
discoverable on
scan on
trust <ev3 address>
pair <ev3 address>
agent on OR default-agent
devices
quit
Device 24:71:89:BA:DA:61 ev3dev
Device 00:16:53:53:64:E1 EV3
It helps to restart Ev3.
The file /etc/bluetooth/rfcomm.conf
rfcomm0 {
# Automatically bind the device at startup
bind no;
# Bluetooth address of the device
device 00:16:53:53:64:E1 ;
# RFCOMM channel for the connection
channel 2;
# Description of the connection
comment "This is Device EV3's serial port.";
}
[To establish Bluetooth] connection, need to create the serial device that binds to the paired Ev3 robot:
sudo rfcomm connect rfcomm0 00:16:53:53:64:E1
or use
sudo rfcomm bind /dev/rfcomm0 00:16:53:53:64:E1 1
where the last number is the communication channel. It needs to be unique.
Some links
- http://www.geekdroppings.com/2018/01/21/raspberry-pi-and-the-lego-ev3-connected-by-bluetooth/
- https://www.hackster.io/KKE/raspberry-pi-lego-mindstorms-ev3-bluetooth-communication-aa42e2
- https://siouxnetontrack.wordpress.com/2013/09/27/connecting-the-pc-to-our-ev3/
- https://gipprojects.wordpress.com/2013/11/29/using-python-and-raspberry-pi-to-communicate-with-lego-mindstorms-ev3/
- https://ev3lessons.com/en/ProgrammingLessons/beyond/EV3PiLight.pdf
- https://ev3lessons.com/en/ProgrammingLessons/beyond/EV3PiCommunicator.pdf
- http://www.abrowndesign.com/2018/10/25/sending-bluetooth-messages-from-raspberry-pi-to-lego-ev3-stock-firmware/
- https://github.com/lehoff/ev3bt/
Transmitting From EV3-G To Raspberry Pi
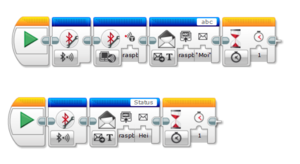
#hackster.io -> Raspberry Pi Lego Mindstorms ev3 bluetooth
import serial
import time
EV3 = serial.Serial('/dev/rfcomm0')
print("Listening to")
try:
while True:
n = EV3.inWaiting()
if n != 0:
s = EV3.read(n)
print( s )
for n in s:
print( "x%02X" % (n), end=' ' )
#print( chr( int(n) ) )
print()
time.sleep(0.5)
except KeyboardInterrupt:
pass
EV3.close()
The received data looks like
b'\x12\x00\x01\x00\x81\x9e\x07Status\x00\x04\x00Hei\x00'
x12 x00 x01 x00 x81 x9E x07 x53 x74 x61 x74 x75 x73 x00 x04 x00 x48 x65 x69 x00
Transmitting From Raspberry Pi To EV3-G
First start the Python script on the Raspberry Pi and then run the EV3 code.
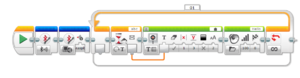
#! /usr/bin/env/ python3
import serial
import time
import EV3BT
EV3 = serial.Serial('/dev/rfcomm0')
s = EV3BT.encodeMessage(EV3BT.MessageType.Text, 'abc', 'Have fun')
for i in range(10):
print(i)
s = EV3BT.encodeMessage(EV3BT.MessageType.Text, 'abc', 'Have fun'+ str(i))
#s = EV3BT.encodeMessage(EV3BT.MessageType.Numeric, 'abc', 123)
#s = EV3BT.encodeMessage(EV3BT.MessageType.Text, 'abc', 'Have fun')
print( EV3BT.printMessage(s) )
EV3.write(s)
time.sleep(1)
EV3.close()
The brick says once Hello' and after that wants to connect to raspi. If the wrong password is given, the Python code will shutdown. The update
in the EV3-G's Bluetooth command do not work.